How to use IP Webcam with opencv as a wireless camera
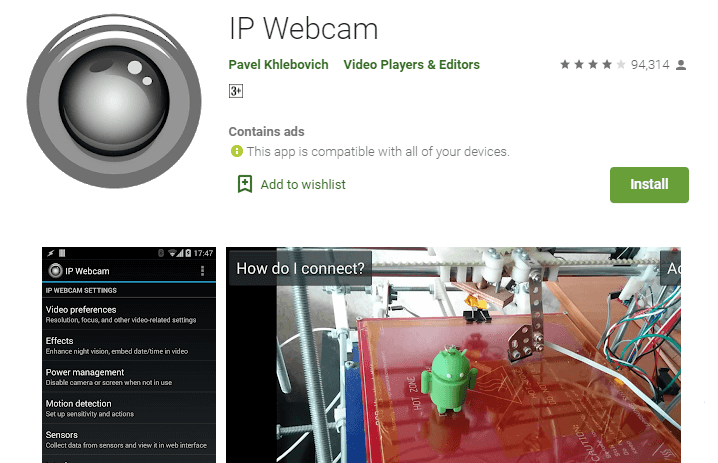
Hi guys, If you are interested in creating robots, or embedded systems like me then you must be wondering if there is an way to use your android phone as an wireless camera (IP Webcam ) for your opencv code embedded in a SBC like Raspberry Pi,
You might have already know about IP Webcam apps for smart phones, but for those who don’t know yet, its an application which streams live images from your mobile camera over local wifi network, and you can see it through web browser.
Okay so now that we all are on same page, lets talk about how we can grab that image streaming on local network in to opencv.
Prerequisites
- IP webCam (from here)
- Android Phone
Setting up IP Webcam
After installing IP webcam in your phone, open it up and you will see an IP address in the screen with a port number like below.
Note that down somewhere, now type that in your browser. it will open up the IP webcam dashboard, which will look like below
Now we need to get the url to get image frames from this dashboard. To do that go to javascript option and we will see the live camera feed from the phone into the browser. just Right click and select “Copy Image Address”
Now paste that link somewhere like a notepad we will be needing that in our code
Lets Code
So now we are done with the setup all we need to do now is write a python code to grab a frame from the url that we noted down in our last step and display that in an infinite loop
lets import the libraries
import urllib
import cv2
import numpy as np
so imported the cv2 for opencv and numpy for matrix manipulation and urllib for opening url and reading values from urls
now we need to read the image from the url that we noted down earlier
url='http://192.168.0.103:8080/shot.jpg'
now its time to construct the loop
while True:
imgResp=urllib.urlopen(url)
imgNp=np.array(bytearray(imgResp.read()),dtype=np.uint8)
img=cv2.imdecode(imgNp,-1)
cv2.imshow('test',img)
if ord('q')==cv2.waitKey(10):
exit(0)
So what just happened up there
Inside the while loop, we are opening the url using “urllib.urlopen(url)” this will give us an response in a variable “imgResp”,
imgNp=np.array(bytearray(imgResp.read()),dtype=np.uint8)
In this line we are reading the values from the url response and converting it to a byte array and ultimately converting it to a numpy array and stored it in a numpy variable imgNp. This is our image. in encoded formate.
img=cv2.imdecode(imgNp,-1)
In this line we decode the encoded image and store it in another variable called img. and thats out final image. Now after that we can do out image processing in that image or what ever we want and then finally display that image we an imshow-waitKey combination
cv2.imshow('test',img)
if ord('q')==cv2.waitKey(10):
exit(0)
Finally all put together
import urllib
import cv2
import numpy as np
url='http://192.168.0.103:8080/shot.jpg'
while True:
imgResp=urllib.urlopen(url)
imgNp=np.array(bytearray(imgResp.read()),dtype=np.uint8)
img=cv2.imdecode(imgNp,-1)
# all the opencv processing is done here
cv2.imshow('test',img)
if ord('q')==cv2.waitKey(10):
exit(0)